题目
给定一个m x
n大小的矩阵(m行,n列),按螺旋的顺序返回矩阵中的所有元素。
示例1
输入:
1
| [[1,2,3],[4,5,6],[7,8,9]]
|
输出:
思路
分析
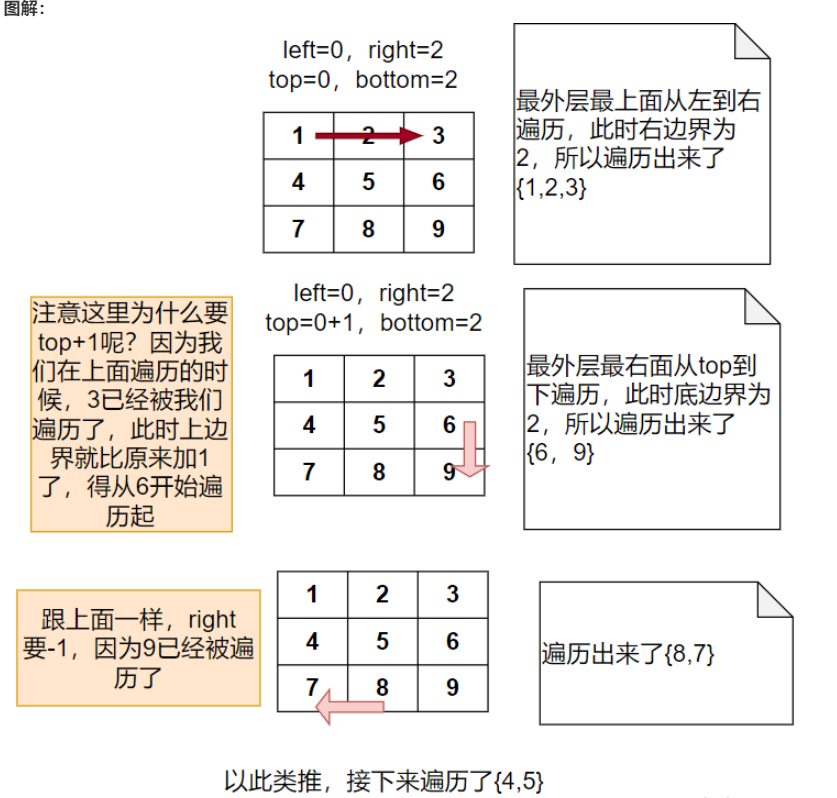
分别需要实现四个遍历:
左→右
上→下
右→左
下→上
需要特别注意的是不要遍历重复数据。left、right、top、bottom的指针变化方式尤为重要。
另外还要注意限制条件top!= bottom 和 left!= right
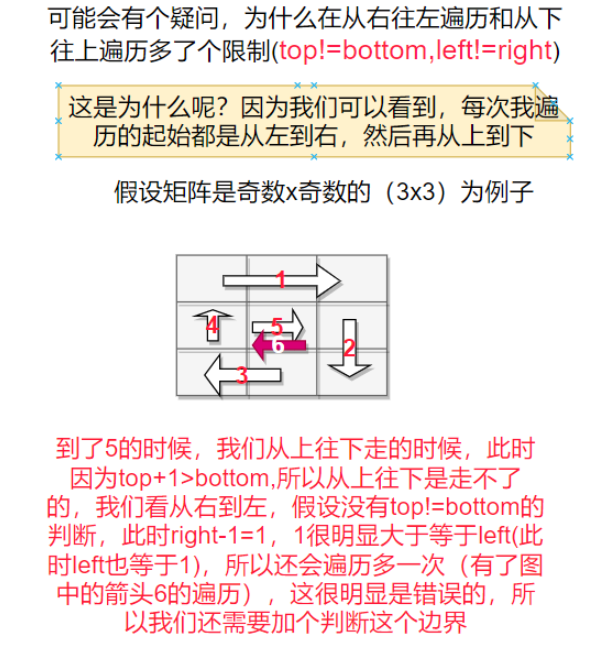
实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| import java.util.*; public class Solution { public ArrayList<Integer> spiralOrder(int[][] matrix) { ArrayList<Integer> res = new ArrayList<>(); if(matrix.length == 0) return res; int left = 0, right = matrix[0].length - 1; int top = 0, bottom = matrix.length - 1; while(top < (matrix.length + 1) / 2 && left < (matrix[0].length + 1) / 2){ for(int i = left; i <= right; i++){ res.add(matrix[top][i]); } for(int i = top + 1; i <= bottom; i++){ res.add(matrix[i][right]); } for(int i = right - 1; i >= left && top != bottom; i--){ res.add(matrix[bottom][i]); } for(int i = bottom - 1; i >= top + 1 && left != right; i--){ res.add(matrix[i][left]); } left++; top++; right--; bottom--; } return res; } }
|